Code Analysis in .NET 5 and 6
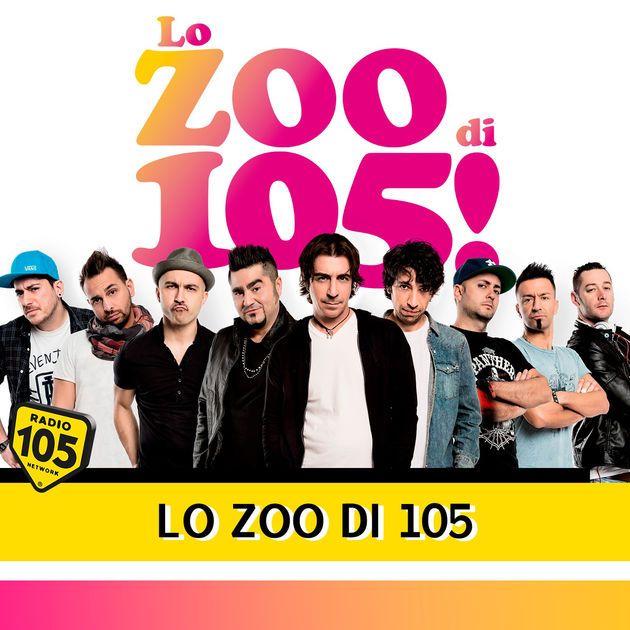
With my last upgrade of the Zoo di 105 podcast from .NET Core 3.1 to .NET 6, I have also updated how code analysis is implemented in the project.
In the previous versions of .NET Core, it was implemented via a NuGet package (initially Microsoft.CodeAnalysis.FxCopAnalyzers and then Microsoft.CodeAnalysis.NetAnalyzers).
Since .NET 5, code analysis is automatically included in the framework, but it is enabled only for a few rules.
Just to be clear: it is still possible to use the "old" system including the NuGet package (and the ruleset file). This has the advantage that you can delay the upgrade to the latest version of the .NET SDK, still using the latest versions of the code analysis (how? simply updating the NuGet package).
But: if you plan to upgrade consistently with the newer versions of .NET, the method that I describe here below is simpler.
It also doesn't use the ruleset files anymore, and this is an improvement, because the ruleset files were long xml files difficult to manage. Now there are much better alternatives.
Enabling Code Analysis
As I've written previously, code analysis is automatically included starting from .NET 5. But only a small subset of rules is enabled by default.
To enable all the available rules, in .NET 6+, add to your projects - or much better, to your Directory.build.props file:
<Project>
<PropertyGroup>
...
<AnalysisLevel>latest-All</AnalysisLevel>
</PropertyGroup>
</Project>
As documented in Configuration options for code analysis, in .NET 6 the AnalysisMode has been replaced with the omni-comprehensive AnalysisLevel, with the suggested value of latest-All.
If you are still on .NET 5, then use the "old" AnalysisMode:
<Project>
<PropertyGroup>
...
<AnalysisMode>All</AnalysisMode>
</PropertyGroup>
</Project>
Disabling Rules
Now the second point: disabling rules.
The official documentation suggests to disable rules in your .editorconfig file.
For example, to disable the rule CA1305, add to your .editoconfig file the following:
dotnet_diagnostic.CA1305.severity = none
In this way, listing only the rules that are disabled, it's much more easy to track them.
But: this method disables only the rules that are file-scoped. There are some rules that are project-scoped, like CA1014.
In this case, the rules can be suppressed in another file, .globalconfig.
Luckily, all rules can be moved into this .globalconfig file, that will have the following format (it contains both file-scoped and project-scoped rules):
# Top level entry required to mark this as a global AnalyzerConfig file
is_global = true
dotnet_diagnostic.CA1014.severity = none
dotnet_diagnostic.CA1305.severity = none
Clearly, this means that you don't need to modify the .editorconfig file at all.
Tooling issues
This new way of implementing Code Analysis brings some regression in the tooling.
I hope that these issues will be solved in a future version of Visual Studio. Meanwhile I have opened this User Voice (feel free to upvote it if you find it helpful):
- With the ruleset file I was used to enable all rules, and this was very easy thanks to the dedicated page in Visual Studio.
Now this is not possible anymore (in a quick way), as we need to enable each rule one by one.
Update: my feedback about multi-select has been moved to this GitHub issue to track it. - Also searching for rules (via code or description, for example like "async" or "dispose") is not possible anymore.
Update: searching is indeed possible, but it's not very visible, because it's over the tabs. A fix is already coming with Visual Studio 2022 version 17.1. See this GitHub issue.
Additional considerations
In this last section, I want to add additional considerations:
- If you want to enable all rules, the already described <AnalysisLevel> (for .NET 6+) and/or <AnalysisMode> (for .NET 5) are enough.
- It's not possible to enable/disable rules per NuGet package.
- It's possible to configure rules per category, as described in MSBuild reference for .NET SDK projects / Code analysis properties.
- Related to the previous point, the list of all built-in categories is documented at Rule categories.
- You can enable all warnings as errors with TreatWarningsAsErrors.
But then, you can disable all code analysis warnings from being treated as errors with CodeAnalysisTreatWarningsAsErrors.
References
Here is the Microsoft official documentation on this topic: