How to configure Code Analysis in .NET Core
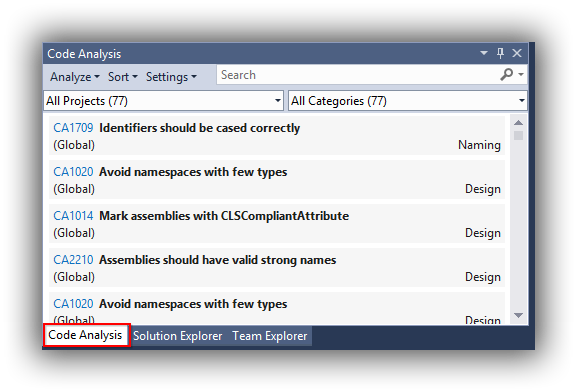
Code Analysis (formerly FxCop) is a very powerful tool to help improving the code quality in .NET projects.
Let's make a bit of history: the previous version of this tool (FxCop) worked analyzing the compiled assemblies, searching for pattern of improvable code, in different areas, like for example performance, globalization, and so on.
With .NET Core, we have a new generation of Roslyn analyzers that work instead during compile time, and even when you type your code in the editor! Additionally, they usually offer code fixes (in Visual Studio: Quick Actions) that help to automatically fix the found issues.
In this post, I will go through its setup, as it is different from the previous FxCop setup.
-
First of all, the biggest difference is how they are included in the projects. You can follow Configure and use Roslyn analyzer rules, but more simply, it's enough to add a NuGet package, Microsoft.CodeAnalysis.FxCopAnalyzers, to all projects of your solution that you want to analyze:
-
Enable Full Solution Analysis from Tools, Options, Text Editor, C#, Advanced, Enable Full Solution Analysis:
-
Create a new RuleSet file at solution level. You can do this right-clicking on the solution itself or on a solution folder and adding a new item of type Code Analysis Rule Set:
-
Edit the rule set file and set all the action for every issue type to Warning.
This should be your starting point; in the next steps, we will remove the rules we don't need. -
Associate each project to the rule file.
To do this, open each project file (right click, Edit.csproj) and add the following tag to a PropertyGroup (what you insert, is the rule set file name, including its path relative to the position of the csproj file itself):
<PropertyGroup>
...
<CodeAnalysisRuleSet>..\RuleSet1.ruleset</CodeAnalysisRuleSet>
</PropertyGroup>
-
Due to a temporary bug, even when you choose to disable a rule at Rule Set level, it's possible that the warnings don't go away. Look for example at the picture here below: I have de-flagged the rule CA1305, but even after a full rebuild, the warning are still there:
So, you need to edit the rule set file manually to disable this rule.
You need to find in which group the rule is defined, and for this you can check the GitHub analyzer repository README. In case you don't find it, I suggest searching by text in all the repository, like this search query.
In particular, from Analyzers_ShippingRules.ruleset of the previous search, we determine the AnalzyerId and the RuleNamespace, to be used in our modification of the rule set file:
<?xml version="1.0" encoding="utf-8"?>
<RuleSet Name="New Rule Set" Description=" " ToolsVersion="15.0">
...
<Rules AnalyzerId="System.Runtime.Analyzers" RuleNamespace="System.Runtime.Analyzers">
<Rule Id="CA1305" Action="None" />
</Rules>
</RuleSet>
-
After have done all of this, recompiling the project will not show the unwanted rules anymore. Here you can see that the rule CA1305 is not there anymore:
Important tip: there is possibly a final optimization, that you can apply if:
- you want to analyze all projects of a solution;
- and all projects relative paths to the rule set file are the same.
If the two conditions are above are satisfied, then you can leverage what described in Customize your build: you can add the NuGet package and configure the rule set file for all projects in the solution, simply creating an external file in the build root folder of your solution.
So, you need to create at the solution level a new file called Directory.Build.props with the following content:
<Project>
<PropertyGroup>
<CodeAnalysisRuleSet>$(SolutionDir)RuleSet1.ruleset</CodeAnalysisRuleSet>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.CodeAnalysis.FxCopAnalyzers" Version="3.3.2" />
</ItemGroup>
</Project>
Following the above hint, here the final order of steps to configure Code Analysis in .NET Core:
- Add at the solution level the file Directory.Build.props with the content shown here above;
- Enable Full Solution Analysis;
- Create a new Rule Set file;
- Edit the Rule Set file, configuring all types of issues to Warning;
- To fix the temporary bug, add the missing rules when needed;
- Rebuild your project.
Update on January 2021
The NuGet package Microsoft.CodeAnalysis.FxCopAnalyzers is now declared obsolete and you should use Microsoft.CodeAnalysis.NetAnalyzers instead.