SwiftUI: Preview in Landscape
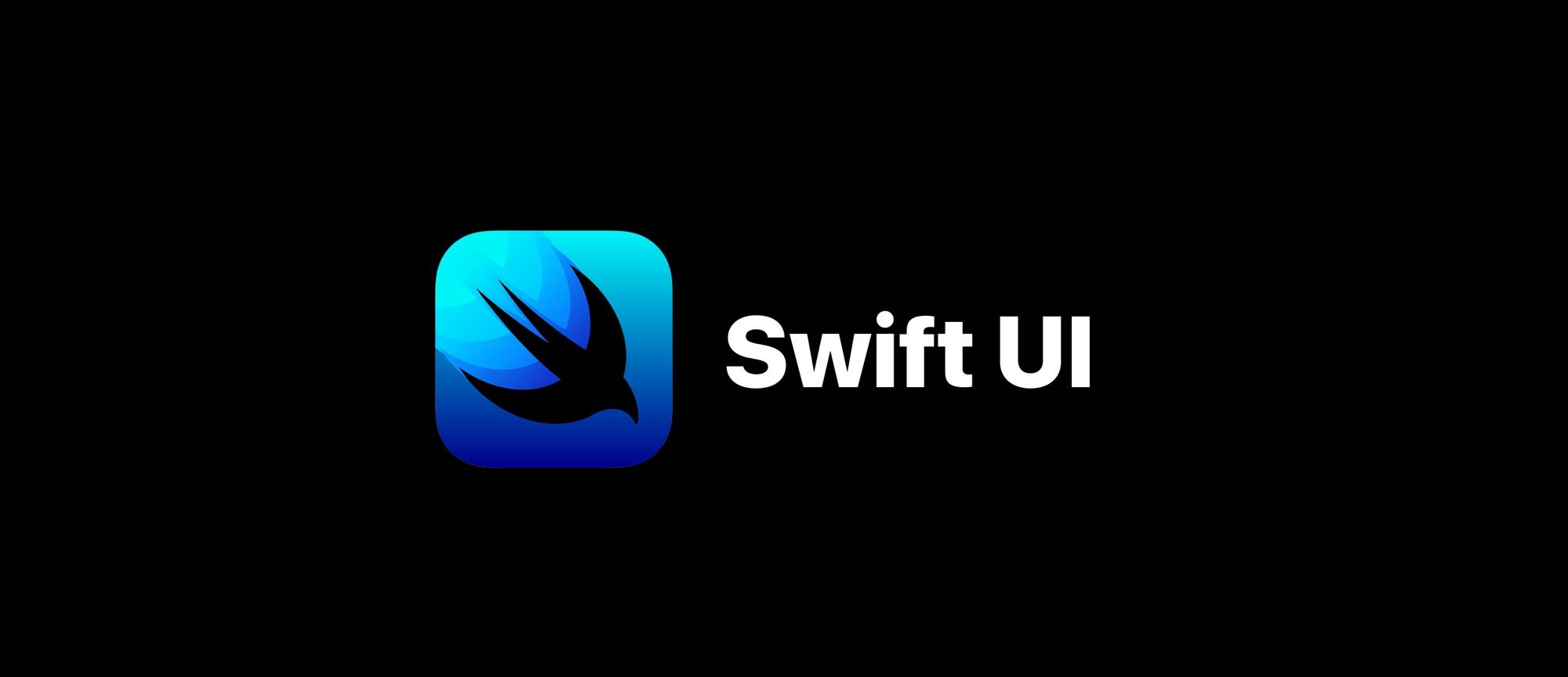
Even in XCode 12, it is still not possible to run a preview in landscape.
I list here two solutions I have found.. but note that with live preview, both options are ignored - we will really need to wait for an update from Apple to definitely solve this issue completely.
First solution
You can simulate it in this way:
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
.previewLayout(.fixed(width: 2532 / 3.0, height: 1170 / 3.0))
.environment(\.horizontalSizeClass, .regular)
.environment(\.verticalSizeClass, .compact)
}
}
You can find the list with all iPhone resolutions at The Ultimate Guide To iPhone Resolutions and iOS Resolution.
Another alternative
When doing more researches on this topic, I have found this article: SwiftUI: Managing Previews.
It gives tips to use the preview in landscape, and also creates two previews, one for light mode and another one for dark mode:
#if DEBUG
struct Landscape<Content>: View where Content: View {
let content: () -> Content
let height = UIScreen.main.bounds.width //toggle width height
let width = UIScreen.main.bounds.height
var body: some View {
content().previewLayout(PreviewLayout.fixed(width: width, height: height))
}
}
struct LightAndDark<Content>: View where Content: View {
let content: () -> Content
var body: some View {
ForEach(ColorScheme.allCases, id: \.self) { colorScheme in
content().environment(\.colorScheme, colorScheme).previewDisplayName("\(colorScheme)")
}
}
}
#endif
Once added these helper functions, you can use them in this way:
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
LightAndDark {
Landscape {
ContentView()
.environment(\.horizontalSizeClass, .regular)
.environment(\.verticalSizeClass, .compact)
}
}
}
}